https://school.programmers.co.kr/learn/courses/30/lessons/42748
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
문제를 풀어서 좋았다.
import java.util.*;
import java.util.stream.*;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
for(int i=0; i<commands.length; i++) {
List<Integer> list = Arrays.stream(array).boxed().collect(Collectors.toList());
List<Integer> sublist = list.subList(commands[i][0]-1, commands[i][1]);
Collections.sort(sublist);
answer[i] = sublist.get(commands[i][2]-1);
}
return answer;
}
}
그런데 고민이 시작되었다.
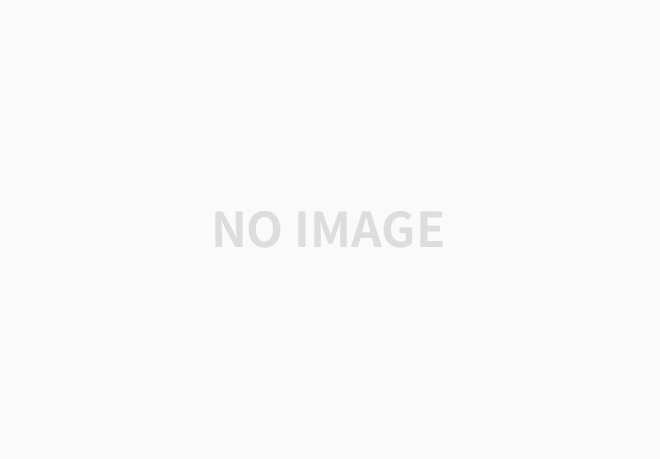
다른 사람의 풀이를 보자...
맨 위에서 두 번째 것.
class Solution {
public int[] solution(int[] array, int[][] commands) {
int n = 0;
int[] ret = new int[commands.length];
while(n < commands.length){
int m = commands[n][1] - commands[n][0] + 1;
if(m == 1){
ret[n] = array[commands[n++][0]-1];
continue;
}
int[] a = new int[m];
int j = 0;
for(int i = commands[n][0]-1; i < commands[n][1]; i++)
a[j++] = array[i];
sort(a, 0, m-1);
ret[n] = a[commands[n++][2]-1];
}
return ret;
}
void sort(int[] a, int left, int right){
int pl = left;
int pr = right;
int x = a[(pl+pr)/2];
do{
while(a[pl] < x) pl++;
while(a[pr] > x) pr--;
if(pl <= pr){
int temp = a[pl];
a[pl] = a[pr];
a[pr] = temp;
pl++;
pr--;
}
}while(pl <= pr);
if(left < pr) sort(a, left, pr);
if(right > pl) sort(a, pl, right);
}
}
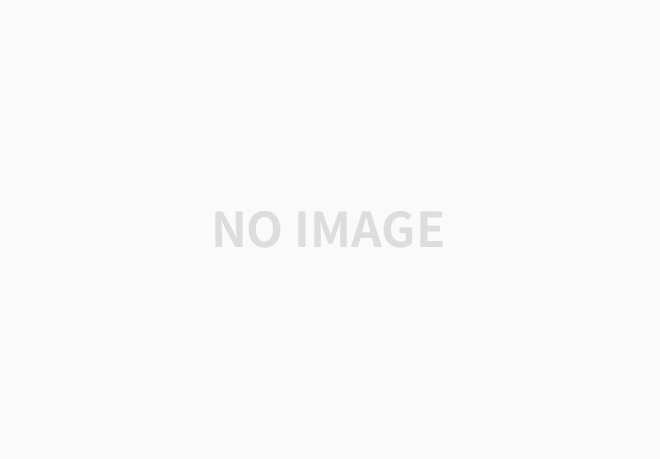
네? 뭐라고요? 100배 차이요?
맨 위의 것.
import java.util.Arrays;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
for(int i=0; i<commands.length; i++){
int[] temp = Arrays.copyOfRange(array, commands[i][0]-1, commands[i][1]);
Arrays.sort(temp);
answer[i] = temp[commands[i][2]-1];
}
return answer;
}
}
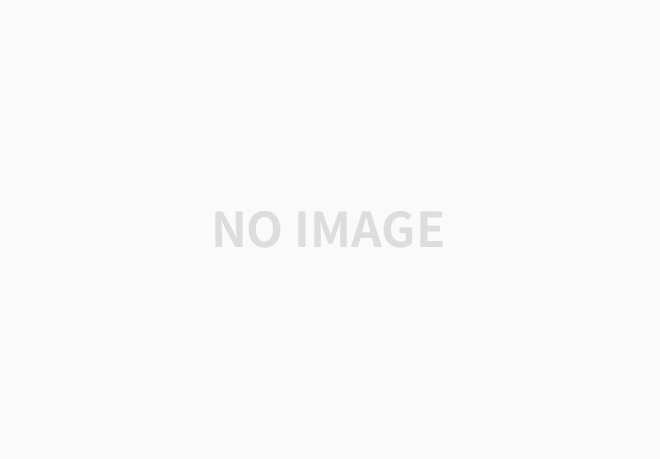
...
일단, 지금 내 수준에서는 두 번째 코드 정도는 절대 구현 못 한다.
세 번째 코드를 읽고 있자니 현타가 왔다.
나 멀쩡한 Array를 왜 List로 변환한 걸까?
아마 Collections.sort() 쓰려고 한 거 같은데
배열은 그냥 Arrays.sort() 쓰면 되잖아..?
Arrays.copyOfRange()도 여러 번 써 봤잖아...?
아-.